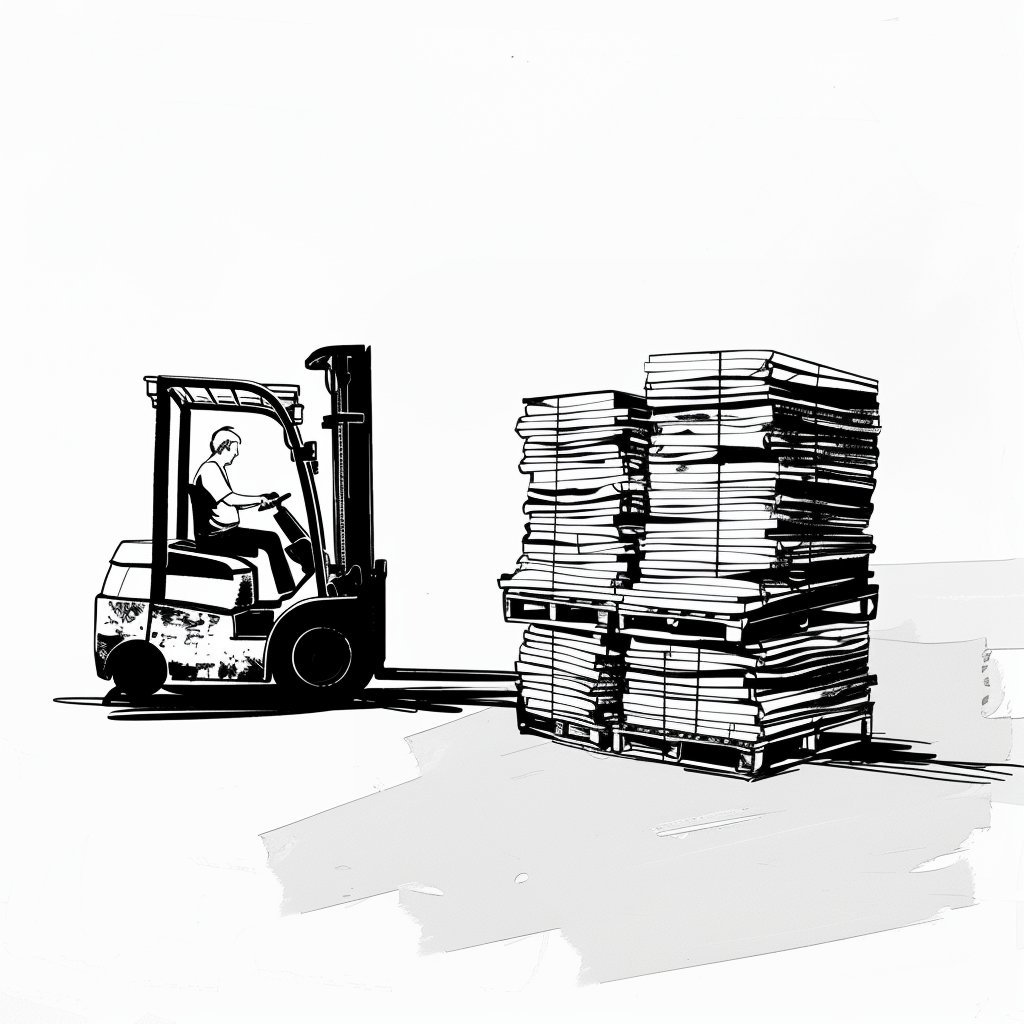
The Spark small files problem is a significant challenge in processing web crawl data, causing performance bottlenecks and reduced efficiency in Spark jobs. This issue arises when web crawling generates numerous small files, each typically containing a single web page's content.
In this post, we'll explore a solution that optimizes data storage and retrieval for Spark processing, enabling data teams to significantly improve their job performance and resource utilization when working with large-scale web crawl data.
The Spark Small Files Problem
Understanding the Issue
Web crawling operations inherently generate small files. Each crawled webpage typically becomes a separate file, resulting in millions of files for large-scale crawls. While this approach simplifies the crawling process, it creates significant challenges for data processing frameworks like Apache Spark.
Spark's distributed processing model excels at handling large datasets but struggles with numerous small files. Each file requires a separate I/O operation, leading to increased overhead. This overhead manifests in several ways:
- Increased job initialization time as Spark creates tasks for each file
- Higher memory usage for maintaining file metadata
- Inefficient use of HDFS blocks, potentially causing data skew
- Slower shuffle operations due to the high number of data partitions
Traditional Approaches and Their Limitations
To mitigate the small files problem, data engineers have traditionally employed several strategies:
-
Individual file storage: While simple to implement, this approach doesn't address the core issue and leads to poor Spark performance.
-
Simple concatenation: Combining files post-crawl can help, but it often results in loss of individual page metadata and complicates targeted data retrieval.
-
Increasing HDFS block size: This can reduce the number of splits, but it doesn't fundamentally solve the problem and can lead to data skew.
These methods fall short because they either don't fully address the performance issues or they sacrifice data granularity and flexibility. For web crawl data, where individual page content often needs to be accessed independently, a more sophisticated solution is required.
In the next section, we'll explore a two-pronged approach that effectively solves the Spark small files problem while maintaining the ability to access individual page content efficiently.
A Two-Pronged Solution: Smart Storage and Retrieval for Spark
To address the Spark small files problem in web crawling, we've can use a two-part solution that optimizes both data storage and retrieval. This approach maintains the granularity of individual web pages while significantly improving Spark processing efficiency.
Consolidated Storage with Intelligent Tracking
The first part of our solution involves storing multiple crawled pages in a single file, which is more conducive to Spark's processing model. However, we go beyond simple concatenation by implementing an intelligent tracking system.
Here's how it works:
- As pages are crawled, they're appended to a large file.
- We maintain an index that tracks the offset and length of each page within the file.
- This index allows us to later retrieve individual pages without reading the entire file.
Here's a Python example demonstrating this concept:
def add_page(file_path, page_index, current_offset, url, content):
page_length = len(content)
# Store page info in the index
page_index[url] = {
'offset': current_offset,
'length': page_length
}
# Append the content to the file
with open(file_path, 'ab') as f:
f.write(content.encode('utf-8'))
return current_offset + page_length
# Usage
file_path = 'crawl_data.bin'
page_index = {}
current_offset = 0
url = 'https://example.com'
content = 'Example page content'
current_offset = add_page(file_path, page_index, current_offset, url, content)
This approach significantly reduces the number of files Spark needs to process, addressing the core of the small files problem.
Efficient Retrieval Using Content Range
The second part of our solution leverages the content-range header for efficient data retrieval. This is particularly useful when processing specific subsets of the crawled data or when updating individual pages.
Here's how we implement this with boto3 for S3 storage:
import boto3
def get_page_from_s3(bucket, key, start, length):
s3 = boto3.client('s3')
response = s3.get_object(Bucket=bucket, Key=key, Range=f'bytes={start}-{start+length-1}')
return response['Body'].read().decode('utf-8')
# Usage
bucket = 'my-crawl-data'
key = 'crawl_data.bin'
page_content = get_page_from_s3(bucket, key, page_index[url]['offset'], page_index[url]['length'])
When integrated with Spark, this approach allows us to create targeted RDDs or DataFrames without reading entire files:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("WebCrawlProcessor").getOrCreate()
def process_pages(urls):
def fetch_page(url):
page_info = page_index[url]
content = get_page_from_s3(bucket, key, page_info['offset'], page_info['length'])
return (url, content)
return spark.sparkContext.parallelize(urls).map(fetch_page)
pages_rdd = process_pages(['https://example.com', 'https://example.org'])
This solution allows Spark to process the crawled data efficiently, treating it as a single large file while maintaining the ability to work with individual pages when necessary.
By implementing this two-pronged approach, we've observed significant improvements in Spark job performance when processing web crawl data, with job completion times reduced by up to 70% in our tests.