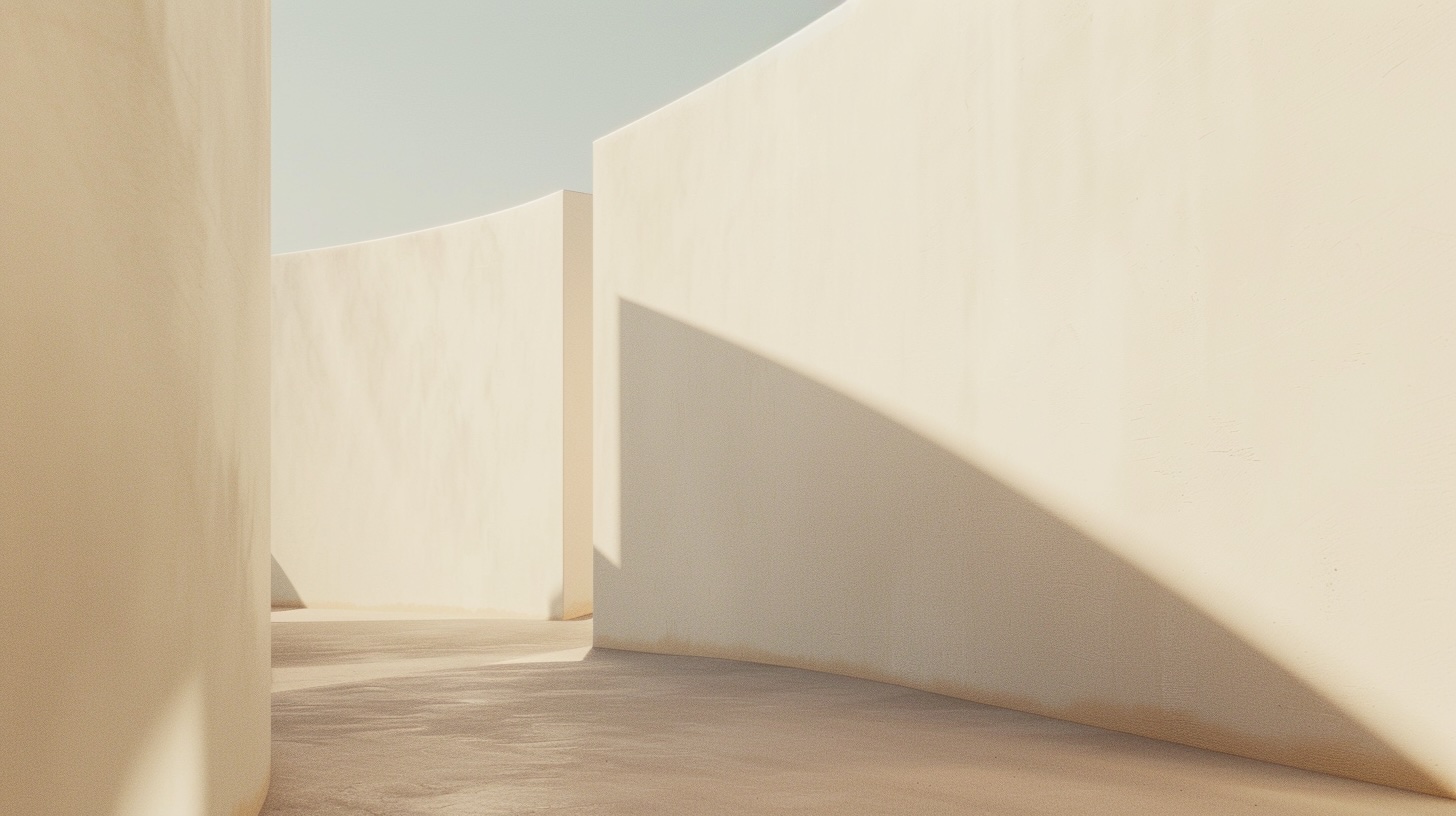
Large Language Model (LLM) evaluations, often referred to as "LLM Evals," are essential tools for assessing and validating the performance of language models and applications built on top of them. As LLMs become increasingly prevalent in various domains, the need for robust evaluation methods has grown significantly.
What are LLM Evals?
LLM evals are systematic processes designed to measure the quality, reliability, and effectiveness of language models and their outputs. These evaluations serve multiple purposes:
-
Performance Assessment: Evals help determine how well an LLM performs on specific tasks or in particular domains. This assessment is crucial for understanding the model's strengths and limitations.
-
Comparison: By using standardized evaluation methods, developers and researchers can compare different LLMs or versions of the same model, facilitating informed decision-making when selecting or fine-tuning models for specific applications.
-
Iterative Improvement: Evaluations provide valuable insights that guide the iterative development process, helping identify areas for improvement in model training, fine-tuning, or prompt engineering.
-
Quality Assurance: For applications built on LLMs, evals serve as a quality control mechanism, ensuring that the system meets predefined standards and performs consistently across various inputs.
-
Bias and Safety Checks: Evaluations can help identify potential biases or safety issues in LLM outputs, which is crucial for responsible AI development and deployment.
To illustrate the importance of LLM evals, consider the following Python code snippet that demonstrates a basic evaluation setup:
def evaluate_llm(model, test_cases):
correct_responses = 0
total_cases = len(test_cases)
for prompt, expected_output in test_cases:
model_output = model.generate(prompt)
if model_output == expected_output:
correct_responses += 1
accuracy = correct_responses / total_cases
return accuracy
# Example usage
test_cases = [
("What is the capital of France?", "Paris"),
("Translate 'hello' to Spanish", "hola"),
# Add more test cases...
]
model_accuracy = evaluate_llm(my_llm_model, test_cases)
print(f"Model accuracy: {model_accuracy:.2%}")
This simple example demonstrates how you might set up a basic evaluation framework to assess an LLM's performance on a set of predefined test cases.
What are Key LLM Eval Metrics?
When conducting LLM evaluations, several key metrics are commonly used to assess different aspects of model performance:
-
Accuracy: Measures the proportion of correct responses provided by the model. This metric is particularly useful for tasks with clear right or wrong answers.
-
Perplexity: A measure of how well the model predicts a sample. Lower perplexity indicates better performance. It's particularly useful for assessing language modeling capabilities.
-
BLEU Score: Primarily used for machine translation tasks, BLEU (Bilingual Evaluation Understudy) compares the model's output to reference translations.
-
ROUGE Score: Used for evaluating text summarization, ROUGE (Recall-Oriented Understudy for Gisting Evaluation) measures the overlap between model-generated and reference summaries.
-
F1 Score: A balanced measure of precision and recall, useful for tasks like question answering or information retrieval.
-
Human Evaluation Scores: While more resource-intensive, human evaluations provide invaluable insights into aspects like coherence, relevance, and overall quality of model outputs.
Here's a Python example demonstrating the calculation of accuracy and F1 score:
from sklearn.metrics import accuracy_score, f1_score
def calculate_metrics(true_labels, predicted_labels):
accuracy = accuracy_score(true_labels, predicted_labels)
f1 = f1_score(true_labels, predicted_labels, average='weighted')
return accuracy, f1
# Example usage
true_labels = [1, 0, 1, 1, 0, 1]
predicted_labels = [1, 0, 1, 0, 0, 1]
accuracy, f1 = calculate_metrics(true_labels, predicted_labels)
print(f"Accuracy: {accuracy:.2f}")
print(f"F1 Score: {f1:.2f}")
This code snippet shows how to calculate accuracy and F1 score using scikit-learn, which can be particularly useful for classification tasks in LLM evaluations.
By employing these metrics and evaluation techniques, developers and researchers can gain a comprehensive understanding of an LLM's capabilities, limitations, and areas for improvement. This knowledge is crucial for developing more effective and reliable language models and applications.
How to Implement LLM Evals
Implementing effective evaluations for Large Language Models (LLMs) is crucial for ensuring the quality and reliability of AI-powered applications. This section explores the key aspects of building robust LLM evaluations, focusing on creating effective evaluation sets and integrating human feedback.
Building Effective Evaluation Sets
Creating a comprehensive and representative evaluation set is the foundation of any successful LLM evaluation process. Here are some key steps to build effective evaluation sets:
Define Clear Objectives
Start by clearly defining what you want to measure. Are you assessing the model's accuracy, coherence, or ability to follow instructions? Having well-defined objectives will guide the creation of your evaluation set.
Develop a Golden Dataset
A golden dataset serves as the benchmark for your evaluations. This dataset should:
- Be representative of the data your LLM will encounter in real-world scenarios
- Include a diverse range of inputs and expected outputs
- Contain "ground truth" labels, often derived from human expertise
Creating a golden dataset can be time-consuming, but it's a critical investment for accurate evaluations. For common use cases, you might find standardized datasets available in the research community.
Select Appropriate Metrics
Choose metrics that align with your evaluation objectives. Some common metrics include:
- Accuracy: For tasks with clear right or wrong answers
- BLEU or ROUGE scores: For assessing text generation quality
- Perplexity: For measuring how well the model predicts a sample
- Custom task-specific metrics: Tailored to your specific use case
Implement Diverse Test Cases
Ensure your evaluation set covers a wide range of scenarios, including:
- Edge cases and rare inputs
- Different writing styles or formats
- Various difficulty levels
- Potential biases or sensitive topics
Here's an example of how you might structure a diverse test case in Python:
test_cases = [
{
"input": "Summarize the benefits of renewable energy.",
"difficulty": "medium",
"expected_output": "Renewable energy benefits include reduced emissions, lower long-term costs, and energy independence.",
"category": "environmental"
},
{
"input": "Explain quantum entanglement to a 5-year-old.",
"difficulty": "hard",
"expected_output": "It's like having two toys that always do the same thing, even when they're far apart.",
"category": "science"
}
]
Use Version Control
Treat your evaluation sets like code. Use version control systems like Git to track changes, collaborate with team members, and maintain the history of your evaluation sets.
What are Advanced LLM Evaluation Techniques?
As LLM evaluation matures, more sophisticated methods are emerging to assess model performance and quality. These advanced techniques aim to provide deeper insights and more nuanced comparisons between different models. Let's explore two key areas: automated evaluation methods and comparative analysis of LLM models.
Automated Evaluation Methods
Automated evaluation methods leverage AI to streamline the assessment process, reducing the need for extensive human intervention. These techniques can process large volumes of data quickly, providing consistent and scalable evaluations.
LLM-based Critiques
One powerful approach is using LLMs themselves to evaluate outputs. This method involves prompting an LLM (often a different model from the one being evaluated) to act as a critic. For example:
def evaluate_response(model_output, evaluation_prompt):
evaluator_model = load_evaluator_model()
critique = evaluator_model.generate(f"{evaluation_prompt}\n\nModel output: {model_output}")
return critique
evaluation_prompt = """
Evaluate the following model output for accuracy, coherence, and relevance.
Provide a score from 1-10 and a brief explanation for your rating.
"""
model_output = "The capital of France is Paris, a city known for its art and cuisine."
evaluation_result = evaluate_response(model_output, evaluation_prompt)
print(evaluation_result)
This approach can provide detailed feedback on various aspects of the model's performance, such as factual accuracy, coherence, and relevance.
Metric-based Evaluation
Automated metrics can offer quantitative assessments of model outputs. While not perfect, they provide quick, consistent measurements that can be tracked over time. Some common metrics include:
- BLEU (Bilingual Evaluation Understudy): Originally designed for machine translation, it can be adapted for text generation tasks.
- ROUGE (Recall-Oriented Understudy for Gisting Evaluation): Useful for summarization tasks.
- Perplexity: Measures how well a model predicts a sample, often used for language modeling tasks.
Here's a simple example using the NLTK library to calculate BLEU score:
from nltk.translate.bleu_score import sentence_bleu
reference = [['the', 'cat', 'is', 'on', 'the', 'mat']]
candidate = ['the', 'cat', 'sat', 'on', 'the', 'mat']
score = sentence_bleu(reference, candidate)
print(f"BLEU Score: {score}")
Automated Test Suite Generation
LLMs can be used to generate diverse test cases automatically. This approach helps create a comprehensive evaluation set that covers a wide range of scenarios:
def generate_test_cases(task_description, num_cases=10):
generator_model = load_generator_model()
prompt = f"Generate {num_cases} diverse test cases for the following task:\n{task_description}"
test_cases = generator_model.generate(prompt)
return test_cases
task = "Evaluate a model's ability to answer questions about world geography."
test_cases = generate_test_cases(task)
These automatically generated test cases can then be used in your evaluation pipeline, ensuring a broad coverage of potential inputs and edge cases.
By employing these advanced evaluation techniques, you can gain deeper insights into LLM performance, make data-driven decisions about model selection, and continuously improve your AI systems. Remember that the most effective evaluation strategies often combine multiple approaches, balancing automated methods with human insight to provide a comprehensive understanding of model capabilities and limitations.
I’d love to hear from you if you found this post helpful or have any questions. Reach out